【Unit Test】使用 Vite + Vitest 進行 React 測試
React + Vitest
首先先下載 Vite + React 的專案
npm create vite@latest
這邊會有兩個問答的選項,我這邊選擇 React + TypeScript + SWC
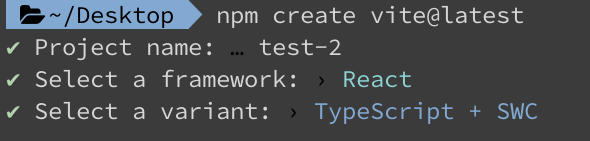
SWC 是一個用 Rust 開發的 JavaScript / TypeScript 編譯器,打包速度比 Babel 還快,現在 Next.js 及 Vite 都有支援 SWC
再來安裝 Vitest
npm i -D vitest
在 package.json 加入 test script
{
"scripts": {
"test": "vitest"
}
}
接著在 vite.config.ts 中加入 reference 跟 test 的設定,設定 globals 為 true,這樣就可以在全域使用 describe
、it
、expect
等方法。
/// <reference types="vitest" />
import { defineConfig } from "vite";
import react from "@vitejs/plugin-react-swc";
export default defineConfig({
plugins: [react()],
test: {
globals: true,
},
});
JavaScript
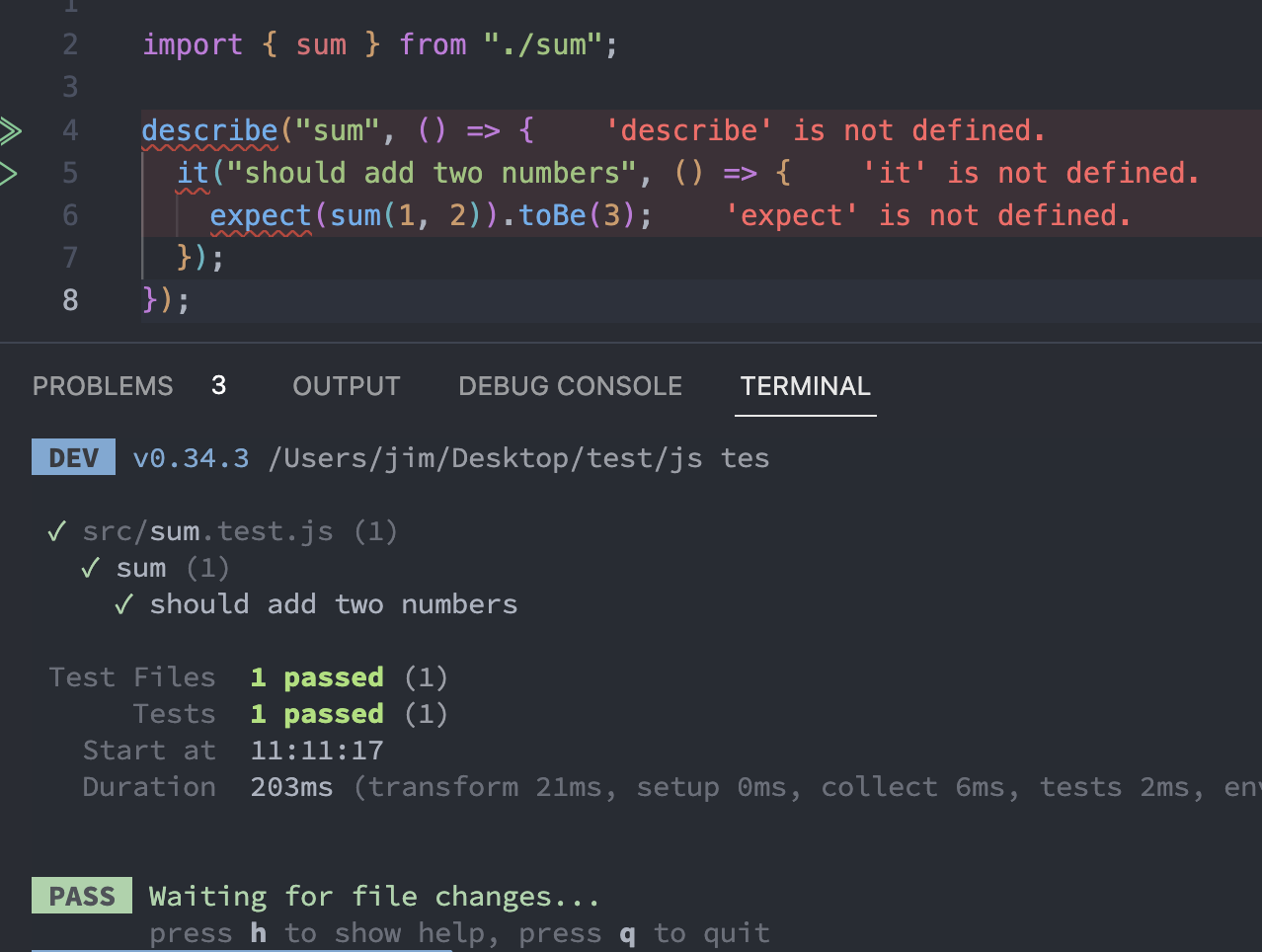
設置完後如果使用 JavaScript 可能會發現會報 eslint 的錯,如上圖,但是跑測試是沒問題的,這是因為 eslint 沒有加入 vitest 的 config,所以會報錯,需要下載 eslint-plugin-vitest 來解決。
npm i -D eslint-plugin-vitest
然後在 eslint 的設定檔中設定 env 及加入 plugin:vitest/globals
。
module.exports = {
env: { browser: true, es2021: true, "vitest/env": true },
extends: [
"eslint:recommended",
"plugin:react/recommended",
"plugin:react/jsx-runtime",
"plugin:react-hooks/recommended",
"plugin:vitest/recommended",
],
};
TypeScript
如果使用 TypeScript 的話,則需要在 tsconfig.json 中加入 types。
{
"compilerOptions": {
"types": ["vitest/globals"]
}
}
React testing library
使用 React 進行測試跟 Jest 一樣加入三個基本的 testing-library 以及測試環境 jsdom。
npm i -D @testing-library/react @testing-library/jest-dom @testing-library/user-event jsdom
JavaScript
建立一個 ./tests/setup.js 檔案把 @testing-library/jest-dom 套件引入,就可以在全域中使用 jest-dom 的擴充語法。
import "@testing-library/jest-dom";
接著在 vite.config.js 加入環境及 setupFile
路徑
/// <reference types="vitest" />
import { defineConfig } from "vite";
import react from "@vitejs/plugin-react-swc";
export default defineConfig({
plugins: [react()],
test: {
globals: true,
environment: "jsdom",
setupFiles: "./tests/setup.js",
},
});
TypeScript
在 TypeScript 因為最近 @testing-library/jest-dom 有進行更版,把 type 型別拉出去了,所以 jest-dom 引入的方式要改成
import "@testing-library/jest-dom/vitest";
然後在 tsconfig.json 把 @testing-library/jest-dom/vitest 加入 types
{
"compilerOptions": {
"types": ["vitest/globals", "@testing-library/jest-dom/vitest"]
}
}